NumPy around() in Python
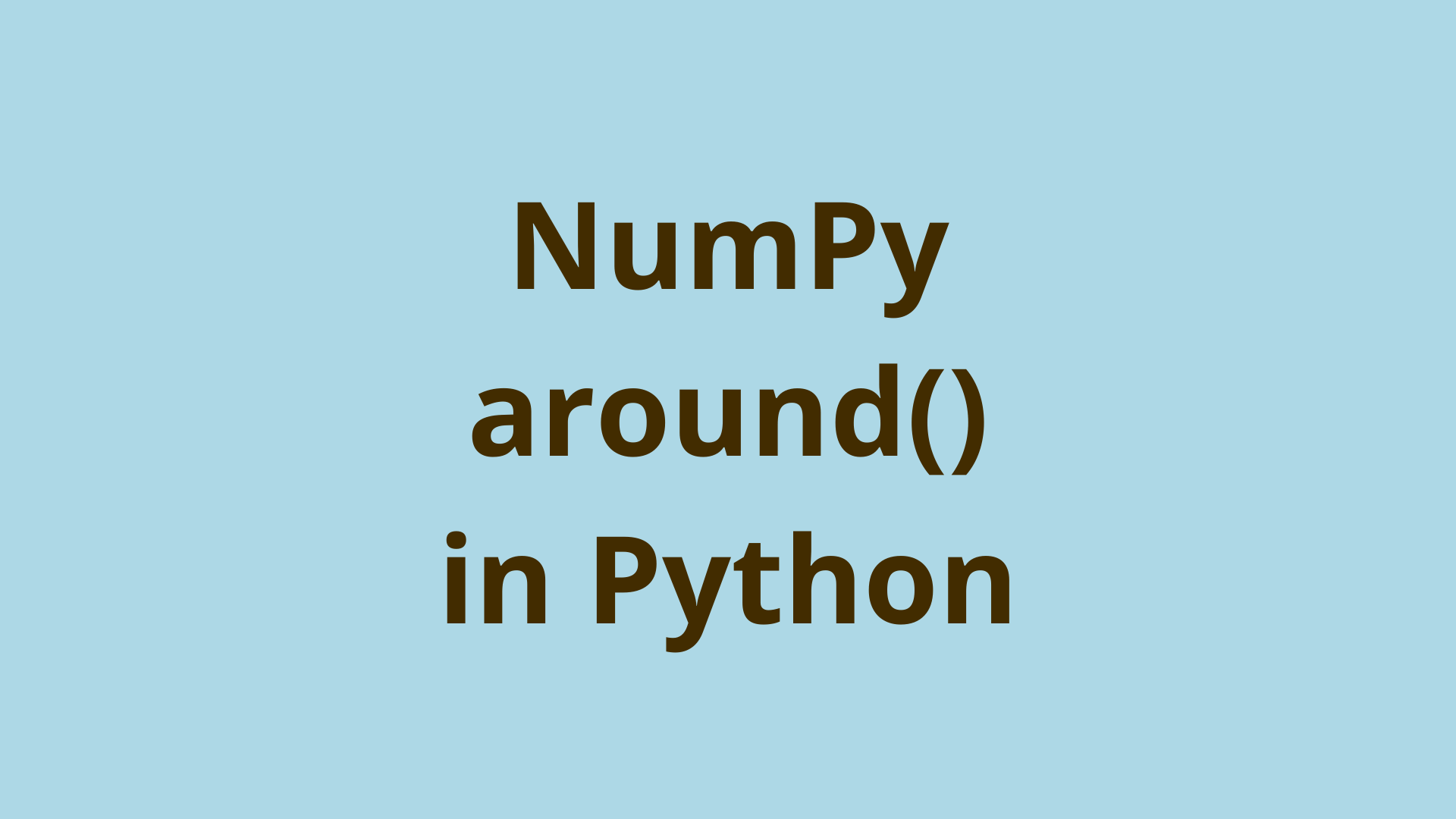
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the NumPy library
- Overview of np.around()
- Various ways to use the decimals argument in np.around()
- np.around() vs round()
- Using np.around() with ndarrays and lists
- Summary
- Next Steps
Introduction
NumPy is a powerful library that adds a new data structure to Python: the n-dimensional array or the ndarray. The ndarray allows for the use of large multi-dimensional arrays and matrices in Python. The NumPy library also contains a variety of functions, which allow us to manipulate and operate ndarray objects.
In this article, we'll discuss the Numpy around()
function (or np around for short) and why it can be better than default options for rounding numbers in Python.
Overview of the NumPy library
Before examining the around() function, it's useful to have a basic understanding of NumPy and its basic usage.
The major advantage of using ndarrays over regular arrays or lists is speed. In fact, ndarrays can be 50x faster than Python built-in lists. Numpy also adds many functions which allow us to easily manipulate these ndarrays. These are just a few of the reasons NumPy is the go-to library in Data Science and Machine Learning, fields in which it is very common to work with large arrays and matrices.
Another small but important detail to mention is when importing NumPy it's often renamed as np
:
import numpy as np
The np.array()
method transforms a regular list into an ndarray:
>>> x = np.array([1, 2, 3])
>>> x
array([1, 2, 3])
>>> type(x)
<class 'numpy.ndarray'>
>>>
You can now see that the array x
is now of the type ndarray. However, NumPy's ndarrays are very similar to Python's lists, in fact, you can use them much the same as a regular Python list.
>>> print(x)
[1 2 3]
>>> for i in x:
... print(i)
...
1
2
3
>>>
Overview of np.around()
Basically, np.around()
is NumPy's function to round floats (decimal numbers) or integers. It takes three arguments:
- The first mandatory argument is the number you want to round
decimals
- optional argument, the number of decimal places you want to round toout
- optional argument, where to output
By default np.around() will round the given number to a the nearest whole number and return the rounded value:
>>> np.around(5)
5
>>> np.around(5.11212)
5.0
>>> np.around(9.11212)
9.0
>>> np.around(9.5)
10.0
>>> np.around(9.49)
9.0
>>> np.around(9.70)
10.0
Above are a couple of examples, which show that np.around() rounds numbers the intuitive way we are used to. Integer inputs remain integers after rounding. If the decimal is below 0.5 the number is rounded down and returns a float. If the decimal is 0.5 or above it rounds up and returns a float.
Various ways to use the decimals argument in np.around()
The decimals
argument allows you to specify what decimal place you want to round the number to. By default, this value is set to 0, and thus every number is rounded to a whole number (the ones decimal place).
If you set this argument to 1 it will round to the tenths place, 2 will round to the hundredths place and so on. For example:
>>> np.around(9.123) # round to ones place
9.0
>>> np.around(9.123, 0) # round to ones place
9.0
>>> np.around(9.123, 1) # round to tenths place
9.1
>>> np.around(9.123, 2) # round to hunredths place
9.12
>>> np.around(9.1234, decimals=3) # round to thousandths place
9.123
>>> np.around(9.1234, decimals=10) # just returns the same number
9.1234
There are a couple of things to note in the example above:
- Leaving out the
decimals
argument or using a value of 0 return the same result. decimals
is the second argument in np.around(), so you can use it with or without thedecimals
keyword.- If a value for
decimals
is entered that exceeds the decimal places of the input number, the same input number is returned.
At this point, a good question to ask would be what happens if you use negative numbers for the decimals
argument? And it's actually pretty important. Negative numbers will allow us to apply rounding in the non-decimal digits of the input number. An argument of -1 will round to the tens place, -2 rounds to the hundreds place, and so on:
>>> np.around(123.12, -1) # round to the tens place
120.0
>>> np.around(123.12, -2) # round to the hundreds place
100.0
>>> np.around(123.12, -3) # round to the thousands place
0.0
>>> np.around(123, -1) # round an integer to the tens place
120
Here you can see that rounding to a decimal place beyond the leftmost digit of the number will result in a 0, and rounding an integer (with negative argument) will always return a whole number.
np.around() vs round()
Python has a built-in function for rounding numbers called round()
and its very similar to np around. By default, it rounds integers or floats to whole numbers, and it even has the decimals
argument as in np.around().
Here are a few comparative examples between the two methods:
>>> round(9) # round to ones place/whole number
9
>>> np.around(9) # round to ones place/whole number
9
>>> round(9.123) # round to ones place/whole number
9
>>> np.around(9.123) # round to ones place/whole number
9.0
>>> round(9.123, 1) # round to tenths place
9.1
>>> round(9.123, 2) # round to hundredths place
9.12
>>> round(123.1, -1) # round to tens place
120.0
>>> round(123.1, -2) # round to hundreds place
100.0
In the above comparisons of round() and np.around(), and it's easy to see that the results are almost the same. But, there are some minor differences:
- When rounding to a whole number, round() returns an integer, while np.around() returns a float.
- When using
round()
, the second argument cannot be used as a keyword argument.
However, the major difference between np.around() and round() is the reason why NumPy is so powerful. With np.around(), you can round all the numbers in entire arrays and matrices.
Using np.around() with ndarrays and lists
The function np.around() is powerful because instead of rounding a single input number at a time, you can specify an ndarray or even a Python list as an argument:
>>> x = [1.1, 2.3, 3.5]
>>> np_x = np.array(x)
>>>
>>> np.around(x) # rounds all the numbers in a list to "ones" place
array([1., 2., 4.])
>>> np.around(np_x) # rounds all the numbers to in ndarray to "ones" place
array([1., 2., 4.])
>>>
Here, x
is a list and np_x
is a ndarray, and we can use both of them as the input for np.around(). This rounds all the numbers in the list and ndarray and returns a ndarray as the output. Of course, you can use the decimals
argument with this too and it will also apply to all the numbers in the arrays.
>>> y = np.array([5.912, 11.123, 1.54])
>>> np.around(y) # rounds all the numbers to ones place
array([ 6., 11., 2.])
>>> np.around(y, 1) # rounds all the numbers to tenths place
array([ 5.9, 11.1, 1.5])
>>> np.around(y, 2) # rounds all the numbers to hundredths place
array([ 5.91, 11.12, 1.54])
>>> np.around(y, -1) # rounds all the numbers to tens place
array([10., 10., 0.])
>>>
One thing to note about np.around() is that it always returns a new ndarray after rounding and doesn't affect the original ndarray (or list). This is where the third argument of np.around(),out
, comes into play. It allows you to specify where the output goes:
>>> y
array([ 5.912, 11.123, 1.54 ])
>>> np_x
array([1.1, 2.3, 3.5])
>>> np.around(y)
array([ 6., 11., 2.])
>>> np.around(y, out=np_x)
array([ 6., 11., 2.])
>>> y
array([ 5.912, 11.123, 1.54 ])
>>> np_x # np_x now stores the output of the previous np.around()
array([ 6., 11., 2.])
Here, you can see that using the out
argument we can send the output of np.around() to np_x
. But, you have to make sure that out
is a ndarray of the same size/shape as the input. The out
argument can allow us to store the output of np.around() back into the original ndarray:
>>> y
array([ 5.912, 11.123, 1.54 ])
>>> np.around(y, out=y)
array([ 6., 11., 2.])
>>> y
array([ 6., 11., 2.])
>>>
Summary
In this article, you looked at the NumPy function around(), understood why it might be better than Python's default options, and understood the overall advantages of using NumPy.
First, you did a short overview of the NumPy library and its basic usage. Second, you saw the np.around() function and its basic usage. Then you did a deep dive on the decimals
argument in np.around() to fully utilize the capabilities of np.around(). Next, you looked at the similarities between Python's built-in function round() and np.around(). Finally, you looked at the major advantage of using np.around() and NumPy and went over the usage of np.around()'s third argument out
.
Next Steps
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers