Sum Function Python
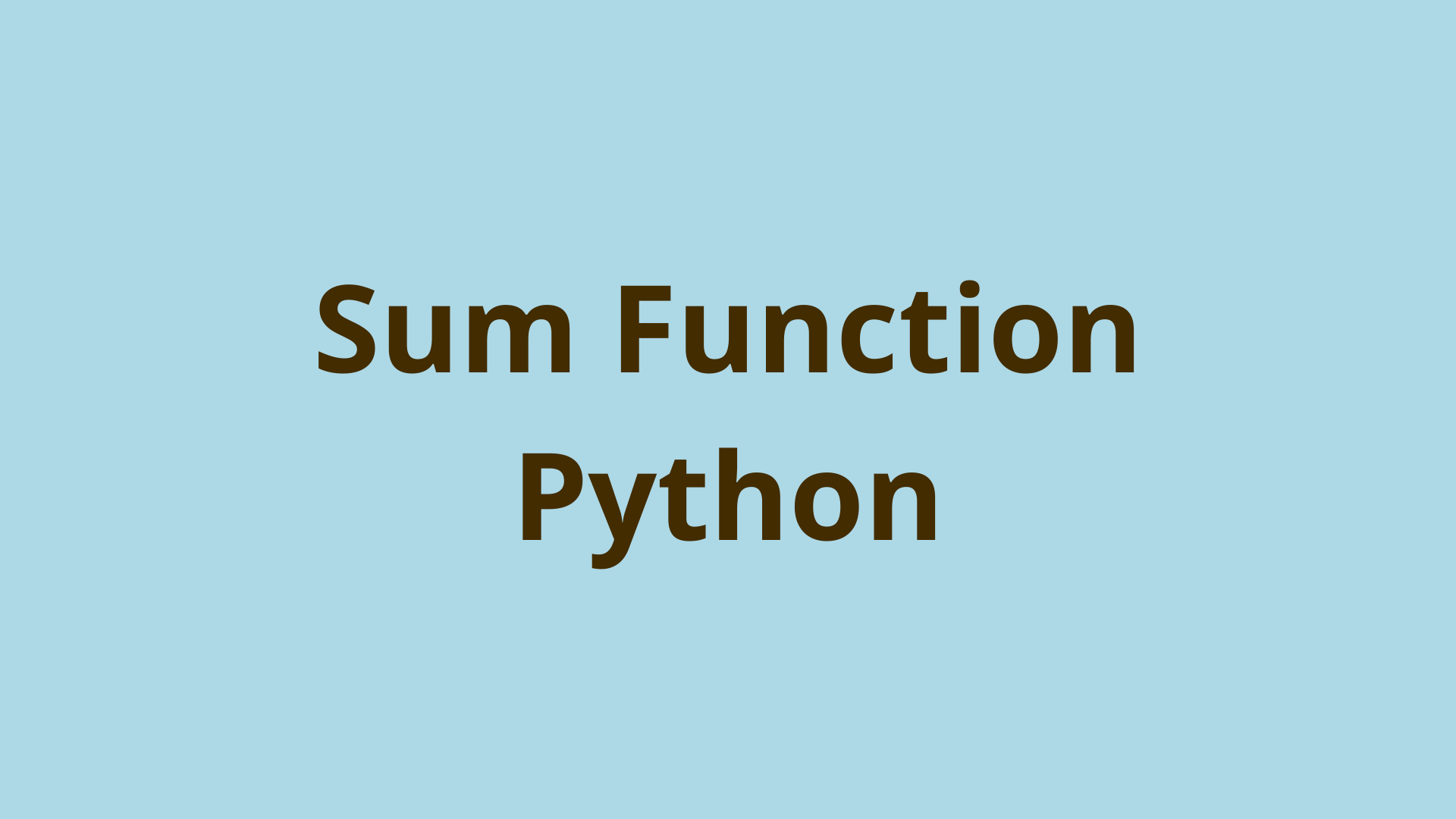
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the Sum Function in Python
- How to Use the Python Sum Function
- Sum a Varying Number of Arguments
- Alternatives to the Sum Function in Python
- Sum a List of Lists
- Summary
- Next Steps
Introduction
Python comes equipped with several built-in functions that allow you to perform basic math operations. This speeds up development time, as there's no need for you to code these operations from scratch. One of the math functions that Python provides you with out of the box is the sum()
function. In this article, you'll get a detailed look at how to use the sum function in Python, as well as how it differs from the plus sign operator (+
).
Overview of the Sum Function in Python
Sum() is a function that takes in an iterable and returns the mathematical sum of its elements. The basic syntax is as follows:
sum(iterable, /, start=0)
The first argument is a positional argument, and it must be an iterable object. Usually, this is a list of numbers, but the sum function in Python will take any iterable as the first argument. The second argument is an optional keyword argument. Note that in the documentation notation, the slash character (/
) separates optional keyword arguments from required positional ones. Here, the keyword argument is the one called start
, an optional starting value from which the sum function should start adding numbers. The default value for this is zero.
As of Python 3.8, the start
argument is a keyword argument that can be defined in the function call itself or elsewhere in the code.
How to Use the Python Sum Function
Let's take a look at several examples that show how to use the sum function in Python.
Sum an Iterable
The most frequent use of the sum function in Python is to sum a list of numbers:
>>> list_to_sum = [1, 2, 3, 4, 5]
>>> sum(list_to_sum)
15
However, lists aren't the only data type that you can sum. The sum function will work with any iterable object that contains numbers. For example, you can sum over a tuple:
>>> tup_to_sum = (1, 2, 3, 4, 5)
>>> sum(tup_to_sum)
15
You can even sum over a dictionary:
>>> dict_with_num_keys = {1: 'a', 2: 'b', 3: 'c'}
>>> sum(dict_with_num_keys)
6
Here, you define a dictionary with numeric values as the keys. When you call the sum function on this dictionary, Python by default will sum all of the keys in the dictionary, as long as they are numeric.
If instead you wanted to sum over the values of a dictionary, then you'd need to explicitly pass those .values()
to the sum function in order to avoid a traceback:
>>> dict_with_num_values = {'a': 1, 'b': 2, 'c': 3}
>>> sum(dict_with_num_values)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
>>> sum(dict_with_num_values.values())
6
When you explicitly pass the .values()
of the dictionary to the sum function, Python will successfully pull the numeric values instead of the keys and return their sum.
Sum From a Start Value
You can specify from what number you want the sum function to start adding numbers with the start
parameter:
>>> sum([1, 2, 3, 4, 5], start=5)
20
This line of code tells Python to sum the numbers one, two, three, four, and five - but to start doing so from the number five. The result is five plus the sum of the list of numbers, which gives twenty.
You don't have to define the start parameter in the function call itself. Because this is a keyword argument, you can define start elsewhere in your code and pass it to the sum function Python call later on:
>>> add_these_numbers = [32, 0, 84, 11, 12]
>>> start_counting_from = 72
>>> sum(add_these_numbers, start_counting_from)
211
This functionality is useful when you have an existing number that you've calculated previously, and you want to continue adding on to it later.
Sum a Varying Number of Arguments
Up until now you've called the sum function with various iterables that contain numeric values. These iterables are usually contained in enclosing characters of some sort (brackets []
for lists, parentheses (``)
for tuples, or curly braces {}
for dictionaries).
However, you may have run into this error if you tried to sum an array of numbers but mistakenly forgot the surrounding brackets:
>>> sum(1, 2, 3, 4, 5)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
Recall that the first argument to the sum function in Python must be an iterable object. Here, you've passed in several numbers separated by commas, but they're not enclosed in any sort of iterable that would link them together. In other words, the first argument passed to the sum function here is 1
, and the number one, an integer object, which is not iterable.
However, there is a way to pass a varying number of arguments to the sum function without explicitly defining them as an iterable beforehand. This requires wrapping the sum function in your own function and using *args
:
>>> def my_sum(*args):
... print(args)
... return sum(args)
...
>>> my_sum(1, 2, 3, 4, 5)
(1, 2, 3, 4, 5)
15
The *args keyword allows you to pass in an argument of varying length to a function. Now, when you call your sum function on a random assortment of numbers, Python first collects them into an iterable object, which it then passes to the sum function from the standard library, before returning the result.
Note that, if you want to also use the start
parameter in your user-defined function, then you'll need to explicitly define it as the first parameter. Otherwise, Python won't recognize the keyword argument:
>>> my_sum(1,2,3,4,5,start=6)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: my_sum() got an unexpected keyword argument 'start'
To use the start
keyword in your wrapper function, define it first:
>>> def my_sum(start=0, *args):
... print(args)
... return sum(args, start)
...
>>> my_sum(1,2,3,4,5)
(2, 3, 4, 5)
15
Now, Python will take the first argument that you pass into your wrapper function and declare it as the start
value. The remaining arguments will be converted to an iterable object and summed together using the sum() function from the Python standard library.
Alternatives to the Sum Function in Python
As you may know, the Python math operators like the plus sign (+
) and multiplication sign (*
) allow you to perform quick operations on various data types. As a result, you might think that the sum function in Python would behave similarly. While this is not quite the case, there are a few simple alternatives you can use to emulate this behavior across data types.
“Sum” a Sequence of Strings
In Python, you can use the plus operator (+
) to add up a sequence of strings:
>>> "P" + "y" + "t" + "h" + "o" + "n"
'Python'
Here, the plus operator is used to combine all of the letters in the word "Python" together.
Now let's see what happens when you try to use the sum function to accomplish the same task:
>>> sum(["P", "y", "t", "h", "o", "n"])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
That raises a traceback error! While the plus operator has no trouble adding strings, the sum function simply presents you with a TypeError
.
There's a similar way to achieve the sum functionality with a sequence of strings, and that's to use the .join
string method:
>>> ''.join(["P", "y", "t", "h", "o", "n"])
'Python'
Here, you tell Python to join together the letters of the word "Python," connecting them with an empty space in between (''
).
You can even wrap this behavior in your own user-defined function:
>>> def string_sum(strings, separator):
... return separator.join(strings)
...
>>> string_sum(["Python", "Java", "C++", "Haskell"], "-")
'Python-Java-C++-Haskell'
The user-defined function string_sum()
behaves similarly to the sum function defined in the Python standard library. It takes in an iterable object - here, a list of strings - and "sums" them up by concatenating them with the given separator in between.
Sum a List of Lists
Like strings, Python also has no trouble adding up a list of lists with the plus operator (+):
>>> [0, 1, 2] + [3, 4, 5] + [6, 7, 8]
[0, 1, 2, 3, 4, 5, 6, 7, 8]
But, just like with strings, you can't use the sum function in Python on a list of lists:
>>> sum([[0, 1, 2], [3, 4, 5], [6, 7, 8]])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'list'
Thankfully, there is an alternative method that you can use. For adding together a list of lists, use the itertools.chain
method instead:
>>> import itertools
>>> itertools.chain([0, 1, 2], [3, 4, 5], [6, 7, 8])
<itertools.chain object at 0x7f403f1b2d00>
>>> list(itertools.chain([0, 1, 2], [3, 4, 5], [6, 7, 8]))
[0, 1, 2, 3, 4, 5, 6, 7, 8]
Note that itertools.chain
returns an object, which you'll need to convert to a list in order to see the final output.
Sum Floating Points More Accurately
Lastly, floating point numbers might not add up to what you'd expect if you use the sum function:
>>> sum([0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1])
0.7999999999999999
This is not a bug, but it's simply the way that floating point numbers work in Python. Tiny imprecisions add up over time as more and more calculations are performed, which can lead to a result that's technically the right answer, but almost certainly not what you'd expect.
This can be especially challenging when you're dealing with things like currencies, which likely require extremely accurate and precise calculations. To mitigate against this, there's a sum function in the Python math module that allows you to more accurately sum floating point numbers:
>>> import math
>>> math.fsum([0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1])
0.8
While the sum function returned an unexpected result, math.fsum()
returns the exact decimal value.
If you want to create a catch-all sum function in Python that handles both integers and floating point values as precisely as possible, then simply wrap fsum() in your own user-defined function:
>>> import math
>>> def my_sum(nums):
... return math.fsum(nums)
...
>>> my_sum([1, 2, 3, 4, 5])
15.0
Now, no matter what kind of numeric values you pass in, the function will return exactly the correct sum.
Summary
In this article, you took a quick look at how to use the sum Python function. You passed in iterable objects of several different types and saw how to specify a starting value. You also looked at how to mitigate against some of the limitations of the sum function in Python, by defining wrapper functions to sum sequences of strings, lists of lists, and floating point numbers.
Next Steps
The sum function is part of the Python standard library. This is a collection of functions and methods that come built-in with your Python installation and are ready for you to use. To see some of the other math operations you can perform out of the box, check out our tutorials on min() and floor().
In this tutorial, you used the sum function to add strings together. Concatenation is only one of many operations you can perform on strings. For example, you can also check to see if a Python string contains a substring.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers