What is AWS CDK? | Building Your First App With AWS CDK
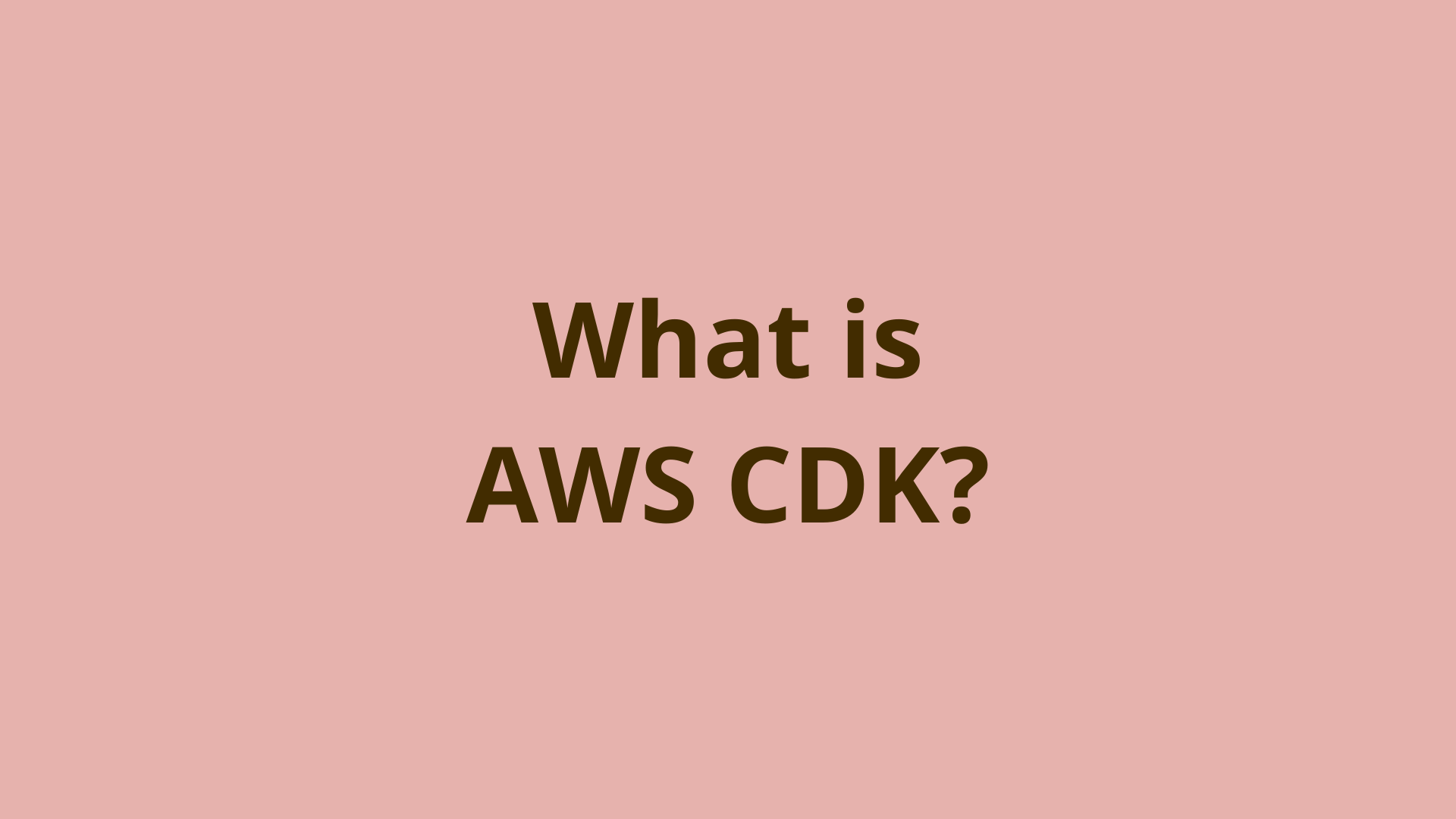
ADVERTISEMENT
Table of Contents
- Introduction
- What is AWS CDK?
- What is the use of AWS CDK?
- What is the difference between AWS SDK and CDK?
- What is the difference between AWS CDK and CloudFormation?
- Getting Started With AWS CDK — AWS CDK Tutorial
- AWS CDK Basic Constructs
- Deploying Your First AWS CDK Application
- Adding More Components | AWS CDK Examples
- Summary
- Next steps
- References
Introduction
When you want to build a backend in the cloud, you have several choices for how to deploy it. While you could manually deploy cloud components via a platform’s GUI or via your command line, it is much easier to do it programatically.
Especially as the complexity of a cloud system or its components increases, it becomes far less manageable to build a system entirely in a GUI or via command line. The solution to this is infrastructure as code (IaC).
What is AWS CDK?
The AWS Cloud Development Kit (CDK) is a software development framework that allows developers to define cloud infrastructure in code and provision it through AWS CloudFormation.
The CDK uses familiar programming languages, including TypeScript, JavaScript, Python, C#, and Java, and deploys everything as a CloudFormation stack. This means that the infrastructure can be easily versioned, shared, and managed, just like application code.
With the CDK, developers can quickly and easily create and manage AWS resources, including EC2 instances, S3 buckets, DynamoDB instances, and Lambda functions. This article will provide a high-level overview of the AWS CDK and its key features.
What is the use of AWS CDK?
Using AWS CDK offers many advantages to developers looking to automate, simplify, and accelerate their cloud development workflow.
- The AWS CDK simplifies the process of defining and provisioning cloud infrastructure by using familiar programming languages. This means that developers can easily understand and work with the code, reducing the potential for human error.
- The CDK allows for greater automation of infrastructure management, making it easier to deploy and update resources as needed. This can save time and effort, and reduce the potential for errors caused by manual processes.
- By using the CDK, infrastructure can be defined and managed as code, allowing it to be versioned, shared, and collaborated on in the same way as application code. This makes it easier to track changes, roll back if necessary, and ensure that the infrastructure is consistent and reliable.
What is the difference between AWS SDK and CDK?
AWS SDK (Software Development Kit) is a collection of libraries, documentation, and sample code that developers can use to build applications that interact with AWS services. The AWS SDK is available in a variety of programming languages, including Java, JavaScript, .NET, PHP, Python, Ruby, and Go, and can be used to build applications for web, mobile, and desktop platforms.
AWS CDK (Cloud Development Kit) is a software development framework for defining cloud infrastructure in code and provisioning it through AWS CloudFormation. The CDK uses familiar programming languages, including TypeScript, JavaScript, Python, C#, and Java, to define cloud infrastructure in a higher-level, more abstract way than CloudFormation templates. This makes it easier for developers to build, deploy, and maintain complex cloud infrastructure.
In a nutshell, the AWS SDK is a toolkit for building applications that interact with AWS services, while the AWS CDK is a framework for defining and provisioning cloud infrastructure using code. They can be used together to build cloud-powered applications, but they serve different purposes and are used at different stages of the development process.
What is the difference between AWS CDK and CloudFormation?
AWS CloudFormation is a service that helps you model and set up your Amazon Web Services resources. You create templates in JSON or YAML to describe the resources you need, and then use the AWS Management Console, AWS CLI, or SDKs to create and manage those resources. CloudFormation enables you to use a template to provision and manage resources in your AWS account.
AWS CDK allows you to build Infrastructure as Code to compile into CloudFormation templates. AWS CDK is a higher-level framework that sits on top of CloudFormation and allows you to define cloud infrastructure using code, while CloudFormation is a service that enables you to create and manage AWS resources using templates. AWS CDK generates CloudFormation templates under the hood, so you can use CloudFormation to manage the resources that are created by AWS CDK.
Getting Started With AWS CDK — AWS CDK Tutorial
In order to use AWS CDK, a user first must have an AWS account and should configure their machine to connect with that account. The AWS Blog has an excellent tutorial on the basic setup for configuring an AWS account on a machine, setting up the cdk
command, and bootstrapping an AWS account to accept CDK-originated resources.
AWS supports many languages for defining CDK components, including TypeScript, JavaScript, Python, Java, C#/.Net, and Go. Of these choices, TypeScript is arguably the most well-documented and widely used, so this tutorial will focus on developing for CDK in TypeScript.
Once you have configured your machine and installed the aws-cdk-lib
dependency, we can create our first CDK app with the following commands.
$ mkdir my-cdk-app
$ cd my-cdk-app
$ cd init app --language typescript
And voila! We now have a very basic CDK app that we can now use for development. Before we continue, let’s quickly review the structure of this app (several basic files have been omitted for brevity).
my-cdk-app/
bin/ -> an entrypoint for the application which loads the definitions from lib
lib/ -> contains stack(s) which will be used to define cloud constructs
my-cdk-app-stack.ts -> **our first stack where we will define constructs**
test/ -> contains tests for the app
cdk.json -> outlines run procedures for the CDK app
tsconfig.json -> contains CDK app's TypeScript configuration
AWS CDK Basic Constructs
CDK relies on code constructs to define cloud components. Every cloud component served by AWS is deployable via a CDK construct.
While the definitions for each type of cloud component may differ, the AWS CDK documentation provides extensive info for the definitions of each component.
We will begin by defining the (arguably) simplest cloud component available on AWS/CDK, the S3 bucket. An S3 bucket can be used for uploading, storing, and retrieving files in the cloud.
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
export class AppStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
const appBucket = new s3.Bucket(this, 'appBucket', {
bucketName: 'app-bucket-name'
});
}
}
Before we deploy our first bucket, let’s break down the code for understanding.
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
In the code above, we are importing aws-cdk-lib
modules. We can use these to express constructs.
const appBucket = new s3.Bucket(this, 'appBucket', {
bucketName: 'app-bucket-name'
});
Now, we use our Bucket
construct to define a new appBucket
object. We can use then reference the appBucket
object later in our infrastructure application to link it with other components.
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
import { PolicyStatement } from 'aws-cdk-lib/aws-iam';
export class AppStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
const appBucket = new s3.Bucket(this, 'appBucket', {
bucketName: 'app-bucket-name'
});
const policyStatement = new PolicyStatement();
policyStatement.addAction('s3:*');
policyStatement.addResource('*');
policyStatement.addAwsAccountPrincipal('<root-account-id>');
appBucket.addToResourcePolicy(policyStatement);
}
}
In this example, we create a new PolicyStatement and add the s3:*
action and *
resource to it, which allows the root AWS account to access all S3 functions. We then add the root account ID as the principal for the policy statement, and add it to the resource policy for the "appBucket" S3 bucket. This will allow the root AWS account to access all S3 functions for the bucket.
Deploying Your First AWS CDK Application
Now that we have our basic app structure, let’s deploy our defined app to an AWS account. We can do this with just two commands.
$ cdk synth
$ cdk deploy
The cdk synth
command creates a Cloudformation template for our app in a newly-generated cdk.out
directory. Then, the cdk deploy
command consumes that template and deploys our components to the cloud.
Depending on the changes you’ve made, this command may ask for permission in the command line to deploy/overwrite cloud components. If you receive this prompt, simply type y
, hit Enter, and continue deployment.
Once cdk deploy
finishes running, it will respond with a new Stack ARN
. An ARN, or Amazon Resource Name, identifies the stack in your account.
Now, go to the Cloudformation module in your AWS Account. From there, you will be able to find the new stack and the components you’ve deployed.
Adding More Components | AWS CDK Examples
With a CDK app, we are probably adding some more components than just an S3 bucket. Here is an example of how you could add a construct for a Lambda function called "MyLambdaFunction" that has read/write privileges on the S3 bucket:
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
import * as lambda from 'aws-cdk-lib/aws-lambda';
import { PolicyStatement } from 'aws-cdk-lib/aws-iam';
export class AppStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
const appBucket = new s3.Bucket(this, 'appBucket', {
bucketName: 'app-bucket-name'
});
const policyStatement = new PolicyStatement();
policyStatement.addAction('s3:*');
policyStatement.addResource('*');
policyStatement.addAwsAccountPrincipal('<root-account-id>');
appBucket.addToResourcePolicy(policyStatement);
const myLambdaFunction = new lambda.Function(this, 'MyLambdaFunction', {
code: lambda.Code.fromAsset('path/to/my/lambda/code'),
runtime: lambda.Runtime.NODEJS_12_X,
handler: 'index.handler'
});
myLambdaFunction.addToRolePolicy(policyStatement);
}
}
To learn more about how a Lambda function works, check out our article AWS Lambda in Plain English.
Summary
In this article, we covered what the AWS Cloud Development Kit (CDK) is and how it can be used to define and manage cloud infrastructure as code. We demonstrated how to create a basic CDK stack, including an S3 bucket, and how to add an account policy statement to the S3 bucket to allow the root AWS account to access all S3 functions. We also showed how to add more CDK constructs, such as a Lambda function, to the stack and give it access to the S3 bucket. By using the CDK, developers can quickly and easily create and manage AWS resources, and easily share, version, and collaborate on their infrastructure as code.
In conclusion, the AWS Cloud Development Kit (CDK) is a powerful tool that allows developers to define and manage their cloud infrastructure as code. With the CDK, developers can quickly and easily create and manage AWS resources, including EC2 instances, S3 buckets, and Lambda functions, and easily share, version, and collaborate on their infrastructure.
So why not take the first step and try the AWS CDK for yourself? Sign up for an AWS account, install the CDK, and start building your infrastructure as code today. Happy coding!
Next steps
If you're interested in learning more about the basics of coding and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Getting Started With CDK, from AWS — https://docs.aws.amazon.com/cdk/v2/guide/getting_started.html
- What is CDK, from AWS — https://docs.aws.amazon.com/cdk/v2/guide/home.html
- CDK Reference Documentation for Constructs — https://docs.aws.amazon.com/cdk/api/v2/
Final Notes
Recommended product: Coding Essentials Guidebook for Developers